gRPC vs REST: Choosing the best API design approach
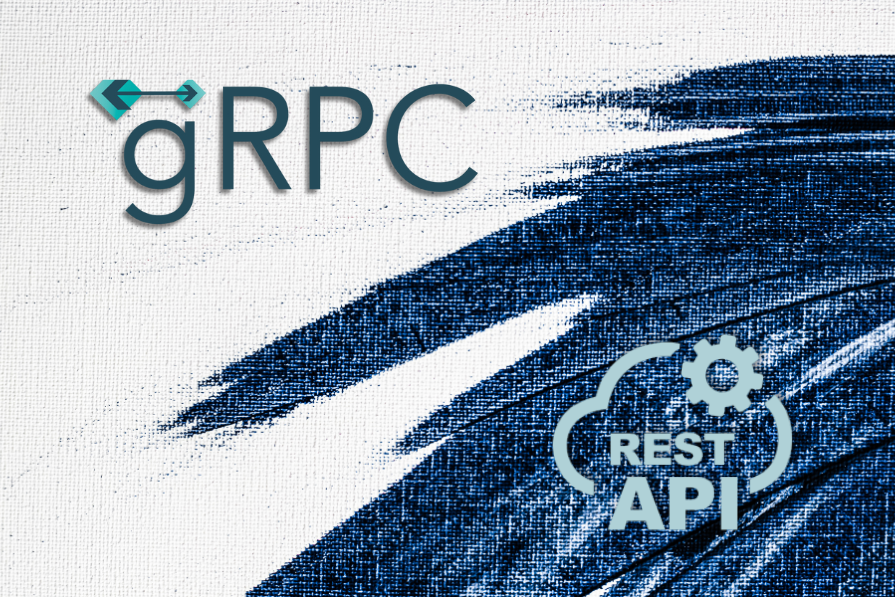
gRPC vs REST: Choosing the best API design approach
TL;DR: Should I choose gRPC or REST?
Choose REST when simplicity, broad compatibility, and human readability are priorities for your application.
Key characteristics of REST
- Follows standard HTTP methods and status codes
- Stateless communications
- Resource-oriented architecture
- Human-readable payloads (typically JSON)
- Widely accepted across browsers and other platforms
Example REST endpoint response
{
"message": "Hello, World!"
}
What is gRPC (gRPC Remote Procedure Call)?
gRPC is Google’s high-performance RPC framework.
Key characteristics of gRPC
- Built on HTTP/2 (multiplexing, server push, header compression)
- Uses Protocol Buffers for efficient binary serialization
- Strong typing through service definitions
- Bidirectional streaming support
- Code generation for multiple languages
Example gRPC service definition
service Greeter {
rpc SayHello (HelloRequest) returns (HelloResponse) {}
}
Under the hood: gRPC and HTTP/2
One of gRPC’s most significant technical advantages is its use of HTTP/2 as a transport protocol. Understanding the technical details of HTTP/2 helps explain why gRPC offers substantial performance benefits.
Technical advantages for gRPC
- Efficient parsing
- Low overhead
- Reduced latency
- Multiplexing
Performance comparison
Serialization and payload size
| | REST | gRPC | |---------------------|------------------------------------|-----------------------------------------------| | Serialization | Uses text-based formats (JSON/XML) | Uses binary Protocol Buffers | | Payload size | Larger due to text encoding | Typically 30-40% smaller than JSON | | Serialization speed | Slower | Faster |
Network performance
- REST primarily uses HTTP/1.1
- gRPC is built on HTTP/2
- Multiplexing in gRPC reduces latency
Benchmarks
In typical scenarios, gRPC outperforms REST in several metrics:
- Throughput
- Latency
- CPU load
- Network utilization
Key advantages comparison
REST advantages over gRPC
- Full browser-level support
- Easy to learn and implement
- Large ecosystem and tooling
- Abundant libraries and middleware
- Extensive documentation
- Well-established security practices
gRPC Advantages Over REST
- Performance
- Strong contract definition
- Streaming capabilities
- Code generation
- Type safety across programming languages
When to choose REST
- Public APIs
- Browser-based applications
- Simple CRUD operations
- Microservices with diverse language needs
- Human-readable debugging
Example REST-based system
When to choose gRPC
- Microservices architecture
- Polyglot environments
- Real-time communication
- Low latency and high throughput systems
- IoT and mobile apps
Example gRPC-based system
Implementation examples
- REST implementation (Node.js/Express)
- gRPC implementation (Node.js)
Final verdict: REST or gRPC?
Here are key factors to consider for your project:
- Client requirements
- Decision flowchart
- Hybrid approaches
- Progressive migration
- Domain-based selection
Conclusion
Both API patterns have their place in modern software architecture. REST, with its simplicity and broad compatibility, remains the go-to choice for public APIs and browser-based applications.