How and when to use type casting in TypeScript
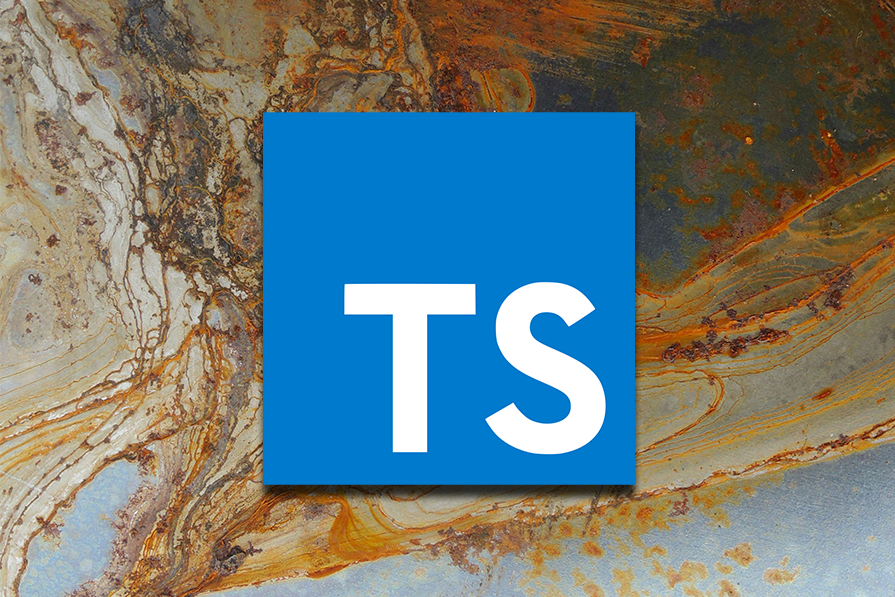
Type Casting in TypeScript
Introduction
- Type casting in TypeScript is a way for developers to tell the compiler to treat a value as a specific type, overriding the inferred type system when the developer has more information than the compiler.
- Foundational concepts like subtype and supertype relationships, type widening, and type narrowing are essential for understanding type casting.
Type Assertion vs Type Casting
- Type assertion: Tell the TypeScript compiler to treat an entity as a different type than it was inferred to be without changing the underlying data type.
- Type casting: Converting a variable from a subtype to a supertype (upcasting) or from a supertype to a subtype (downcasting).
Type Casting with the as
Operator
- The
as
operator is TypeScript's primary mechanism for explicit type casting.
- Example:
const result = (object as SpecificType);
Examples of Type Casting
- Casting to a specific class type using the
as
operator.
- Casting to a string in a function.
- Casting to a union type for expressing multiple possible types.
When to Use Type Casting in TypeScript
- Working with third-party libraries that return loosely typed data.
- Parsing JSON with known structure ahead of time.
- When TypeScript can't infer a more specific type from a generic type.
- Interacting with the DOM or external APIs in browser environments.
Benefits of Type Casting
- Helps with TypeScript's static type system.
- Prevents runtime errors by providing clear types upfront.
- Increases code readability and maintainability.
Conclusion
- Type casting in TypeScript is a powerful tool for handling type mismatches and ensuring type safety in your code.
- Proper use of type casting can help you work with real-world data effectively and avoid potential runtime issues.