How to use the ternary operator in JavaScript
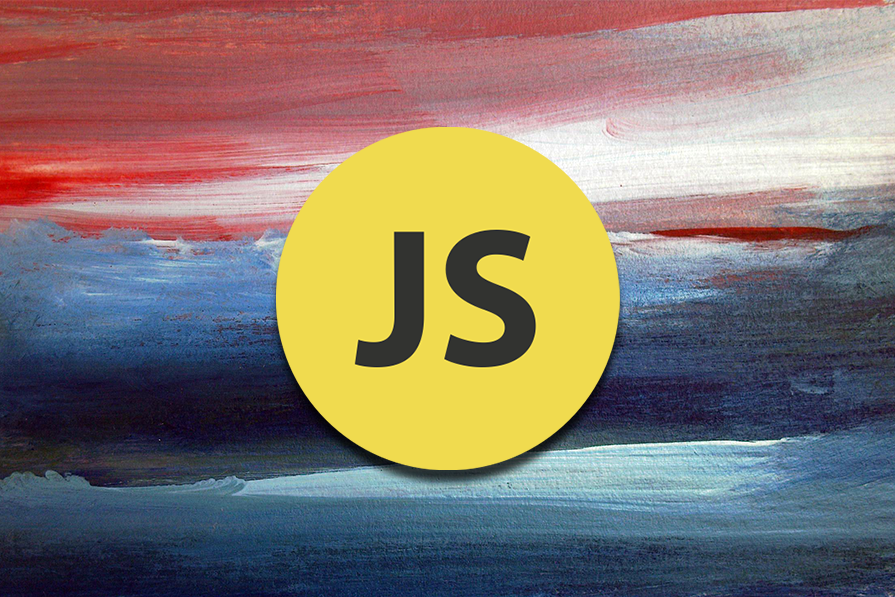
Ternary Operator in JavaScript
The ternary operator in JavaScript is a shorthand way of writing conditional statements. It helps make code cleaner and more concise by condensing multiple lines of code into a single line. It consists of a condition followed by a question mark, the code to execute if the condition is true, a colon, and the code to execute if the condition is false.
Basic Usage of Ternary Operator
- The ternary operator can be used to assign values based on a condition in a compact way.
- It reduces the number of lines of code and improves readability.
- Example:
let message = age >= 14 ? "You are eligible" : "You are not eligible";
Nested Ternary Operators
- Nested ternary operators can be used for multiple conditions in a single line of code.
- It simplifies code and makes it easier to read.
- Example:
let ticketType = age >= 65 ? "Senior" : (age >= 18 ? "Adult" : "Child");
Comparing Ternary Operator with Traditional Statements
- Ternary operators are more concise and readable for simple conditional assignments.
- They can be easier to understand and maintain compared to traditional if-else statements.
- Example:
- Traditional:
if (isLoggedIn) { message = "Welcome back!"; } else { message = "Please log in."; }
- Ternary:
let message = isLoggedIn ? "Welcome back!" : "Please log in.";
- Traditional:
Pros and Cons of Ternary Operator
-
Pros:
- Suitable for complex logic with multiple conditions.
- Ideal for concise and straightforward conditions.
- Improves code readability and maintainability.
-
Cons:
- Can become unreadable when multiply-nested.
- Feels verbose for very simple conditions.
Overall, the ternary operator in JavaScript is a powerful tool that can enhance the clarity and structure of your code, especially when dealing with straightforward conditional assignments.