Integrating Next.js and SignalR to build real-time web apps
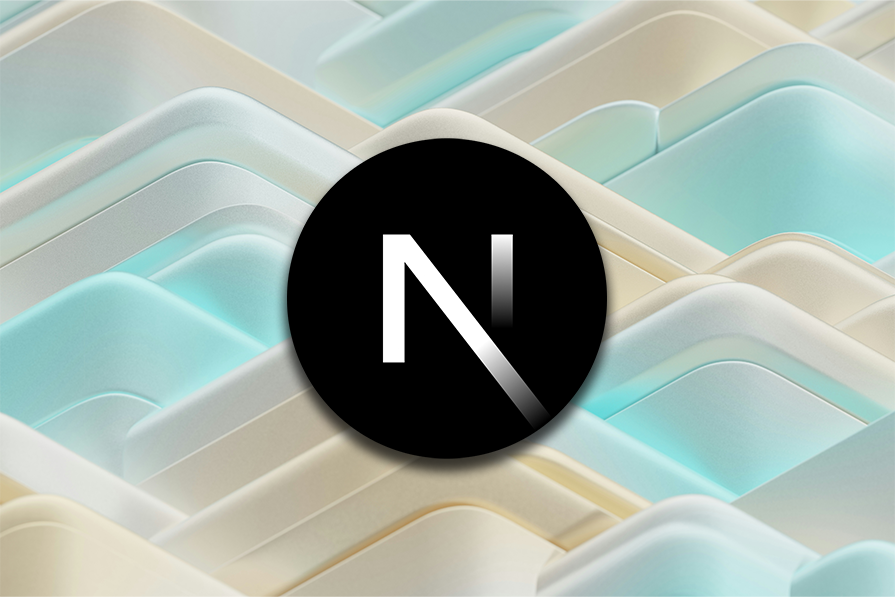
Tutorial: Integrating Next.js and SignalR for Real-Time Web Apps
In this tutorial, we will learn how to integrate Next.js and SignalR to build real-time web applications. SignalR is a powerful tool for building real-time apps with features like WebSockets and intercommunication between clients and servers. Next.js, on the other hand, offers a modular approach and streamlined updates for building performant web apps.
Why Developers Use SignalR
- Easily build real-time web applications with little overhead
- Uses WebSocket protocol for real-time communication
- Enables intercommunication between client and server
- Abstracts complexities of real-time app setup
- Scalable for large apps with frequent updates
Benefits of Using Next.js with SignalR
- Streamlined updates with React's state management
- Modular approach aligns with real-time data streams
- Enhanced real-time features for messaging and live updates
- Cross-platform reach for different browsers and large user bases
Integrating Next.js and SignalR
- Build an ASP.NET Core signal server
- Create a Next.js frontend for real-time chat functionality
- Establish WebSocket methods for sending and receiving messages
- Synchronize state using React Hooks for real-time communication
- Ensure scalability with SignalR for handling concurrent users and requests
By integrating Next.js and SignalR, developers can build scalable, performant, and user-friendly real-time web applications across different platforms.
Conclusion
Combining Next.js and SignalR offers a powerful solution for building real-time web apps with enhanced features and scalability. Whether it's for chat apps, live dashboards, or collaborative platforms, this integration provides a robust foundation for modern web development.
Code Snippets
Below are key code snippets for integrating Next.js and SignalR:
// Initialize SignalR connection in Next.js
const connection = new HubConnectionBuilder()
.withUrl("http://localhost:5000/chat")
.build();
// Send message to server
const sendMessage = (message) => {
connection.invoke("SendMessage", message);
};
// Component to handle real-time chat in Next.js
const ChatApp = () => {
const [messages, setMessages] = useState([]);
useEffect(() => {
connection.start();
connection.on("ReceiveMessage", (message) => {
setMessages([...messages, message]);
});
}, []);
// Method to send new message
const handleSendMessage = (message) => {
sendMessage(message);
};
return (
<div>
{/* Chat UI code here */}
</div>
);
};
By following these steps and utilizing the provided code snippets, developers can seamlessly integrate Next.js and SignalR for building real-time web applications.