Natively implement dynamic imports in React Native
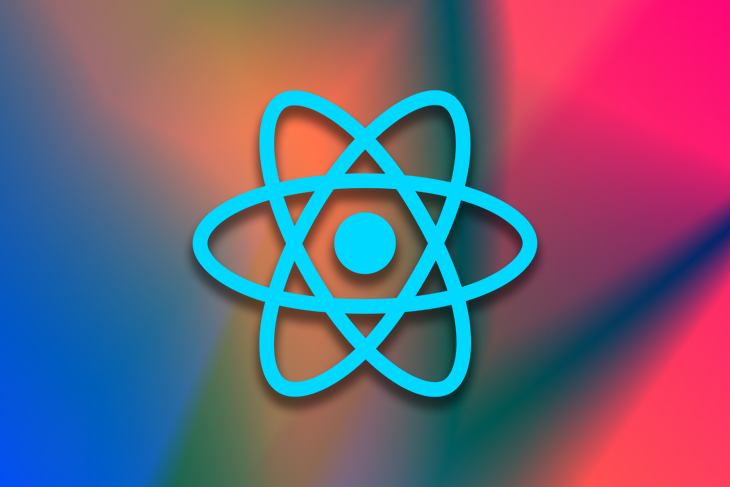
Dynamic Imports in React Native
Dynamic imports allow you to load modules or components in your React Native application only when they are needed, improving the startup time and overall performance of your app. In this article, we will explore how to natively implement dynamic imports in React Native.
Static vs. Dynamic Imports
Static imports, declared at the top of a file using the import
statement, are the traditional way of including modules in JavaScript. On the other hand, dynamic imports allow you to load modules or components at runtime, enabling more efficient code splitting and lazy loading.
How to Natively Implement Dynamic Imports in React Native
To use native dynamic imports in React Native, you need to have React Native version 0.72 or higher installed. There are two approaches to implementing dynamic imports: using the syntax or using the createAsyncComponent
method in the Metro bundler.
Using the Syntax
You can use the dynamic import syntax like this:
function MyComponent() {
const [module, setModule] = useState(null);
useEffect(() => {
import('./MyModule').then((module) => {
setModule(module.default);
});
}, []);
if (!module) {
return null;
}
return <module />;
}
Note: You need to use the await
keyword inside an async
function to wait for the promise to resolve.
Using the createAsyncComponent
method
The createAsyncComponent
method is a supported feature of the Metro bundler that allows you to create a context for dynamic imports. You can use the method to create a context like this:
const MyAsyncComponent = createAsyncComponent(() => import("./MyComponent"));
function App() {
return (
<MyAsyncComponent fallback={<ActivityIndicator />} />
);
}
The first argument of the method is the base directory where you want to look for modules or components.
Third-party Solutions to Achieve Dynamic Imports
If you prefer to use built-in React features, you can use React.lazy()
and Suspense
. React.lazy()
allows you to render components lazily, while Suspense
is used to show a fallback component while the lazy component is loading. Here's an example:
const MyLazyComponent = React.lazy(() => import("./MyComponent"));
function App() {
return (
<Suspense fallback={<ActivityIndicator />}>
<MyLazyComponent />
</Suspense>
);
}
Another popular option is to use the Loadable Components
library. This library provides a simple API to dynamically load and render components. Here's an example:
import Loadable from 'react-loadable';
const MyDynamicComponent = Loadable({
loader: () => import("./MyComponent"),
loading: LoadingComponent,
});
function App() {
return (
<MyDynamicComponent />
);
}
Benefits of Dynamic Imports in React Native
Dynamic imports offer several advantages in React Native:
- Faster startup times: By loading only the required code on demand, dynamic imports can significantly reduce the time it takes for your app to start up, leading to smaller bundle sizes and improved performance.
- Improved code splitting: Dynamic imports enable more efficient code splitting, allowing you to load only the necessary code for each screen or component.
- Lazy loading: Dynamic imports enable lazy loading, which can improve the user experience by loading components as they are needed, rather than all at once.
Best Practices for Using Dynamic Imports
To use dynamic imports effectively in React Native, consider the following best practices:
- Use dynamic imports sparingly: While dynamic imports can improve performance, they are not a silver bullet. Use them selectively for components that are not immediately needed to avoid unnecessary complexity.
- Utilize built-in components and libraries: React Native provides built-in components like
ActivityIndicator
and libraries likeReact.lazy()
andLoadable Components
that make implementing dynamic imports easier and more efficient. - Handle errors and failures: Use error boundaries and fallback components to handle errors and failures when using dynamic imports. This ensures a smooth user experience and prevents the app from crashing.
In conclusion, dynamic imports are a powerful feature that can improve the performance and user experience of your React Native application. By selectively loading code only when needed, you can reduce startup times and optimize the overall performance of your app.