Optimizing build performance in Next.js
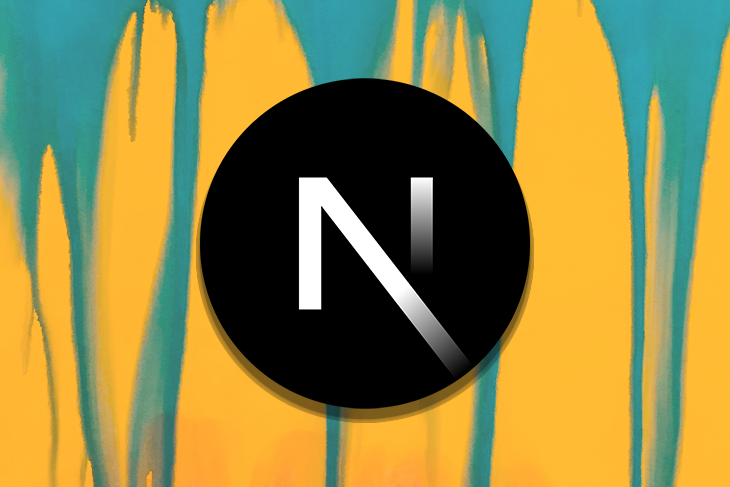
Next.js Performance Optimization
Build performance refers to the time it takes to compile and generate the static files for your application. Slow build performance can negatively impact the overall performance and user experience of your application. Next.js provides several inbuilt optimizations to improve build performance, and there are additional approaches you can take to further optimize your application.
Leveraging Next.js Inbuilt Optimizations
Next.js provides several inbuilt optimizations to improve build performance:
Automatic Static Optimization
If your application does not have dynamic routes, Next.js will automatically render it as static HTML. This means that only the code needed for the current page is loaded on the initial page load, improving performance.
Automatic Image Optimization
Next.js provides the Image
component, which automatically optimizes images with lazy loading and resizing/compression based on device size. This reduces image loading time and improves app performance.
Automatic Font Optimization
Using Next.js, you can load fonts asynchronously using the next/head
component. This reduces the time it takes to load fonts and improves app performance.
Automatic Prefetching
Next.js enables automatic prefetching of pages for faster navigation. This means that while a user is on a page, Next.js will preload the code for the next page, reducing the wait time when navigating to a new page.
Optimizing Third-Party Scripts
To improve performance, you can optimize how third-party scripts are loaded in your application. This can include loading scripts asynchronously, deferring their execution, or using a content delivery network (CDN) to improve the delivery speed.
Optimizing Critical Rendering Path (CRP)
Optimizing the CRP is essential for improving initial load time and perceived performance. This can include reducing the amount of JavaScript executed on the main thread, using async/defer attributes for script tags, and minimizing render-blocking CSS.
Large Contentful Paint (LCP) Optimization
Improving LCP is crucial for a better user experience. Common techniques include optimizing images, reducing render-blocking CSS, and deferring non-critical JavaScript execution.
Using the Intersection Observer API
The Intersection Observer API allows you to observe changes in the intersection of a target element with its ancestor or the viewport. This is useful for lazy loading images and other content that is not immediately visible on the screen, improving performance.
CSS and JS Optimization and Minification
Optimizing and minifying CSS and JavaScript files reduces their file size, leading to faster loading times for your application.
Improving Network Performance
Optimizing network performance involves techniques such as using HTTP/2, reducing the number of requests, compressing files, and leveraging browser caching to improve loading times.
Caching
Implementing caching at various levels (browser, server, CDN) can greatly improve application performance by serving cached content instead of making expensive server requests.
CDN Integration
Using a content delivery network (CDN) can significantly improve the delivery speed of static assets, reducing latency and improving performance for users around the world.
Using Bundle Analysis
Next.js provides tools for analyzing the size and contents of your application bundles. This allows you to identify and optimize large or unnecessary dependencies, reducing the overall bundle size and improving build performance.
Leveraging Dependency Management
Review your project's dependencies and remove any unused or unnecessary packages. This helps reduce the size of the final build and improves build performance.
Removing Unused Dependencies
Remove any unused dependencies or packages from your project as they increase the bundle size unnecessarily and may negatively impact build performance.
Tree Shaking
Next.js uses tree shaking to eliminate unused code from your JavaScript bundle. Make sure your application's code is properly composed to take advantage of this optimization.
Specific Imports
Instead of importing entire modules or libraries, only import the specific parts you need. This reduces the size of the final bundle and improves build performance.
Excluding Unnecessary Files or Folders
Use Next.js configuration options to exclude any unnecessary files or folders from the build process. This ensures that only essential files are included, reducing build time and improving performance.
Next.js Performance Optimization Example
By leveraging Next.js inbuilt optimizations, optimizing the critical rendering path, using the Intersection Observer API, and employing other optimization techniques, you can significantly improve the build performance and overall performance of your Next.js application. Regularly monitoring and analyzing your application's performance with tools like Lighthouse, WebPageTest, and Next.js bundle analysis can help identify areas for further optimization.